C++ Basic Syntax
C++ is a general-purpose, object-oriented programming language. It was developed in 1979 by Bjarne Stroustrup at AT & T Bell Laboratory. It is a high-level programming language & advanced version of C programming language. As Compared to other programming languages like Java, and Python, it is the fastest programming language.
What is Syntax?
Syntax refers to the rules and regulations for writing statements in a programming language. They can also be viewed as the grammatical rules defining the structure of a programming language.
The C++ language also has its syntax for the functionalities it provides. Different statements have different syntax specifying their usage but C++ programs also have basic syntax rules that are followed throughout all the programs.
Basic Syntax of a C++ Program
We can learn about basic C++ Syntax using the following program.
.png)
The image above shows the basic C++ program that contains header files, main function, namespace declaration, etc. Let’s try to understand them one by one.
1. Header File
The header files contain the definition of the functions and macros we are using in our program. They are defined on the top of the C++ program.
In line #1, we used the #include <iostream> statement to tell the compiler to include an iostream header file library which stores the definition of the cin and cout methods that we have used for input and output. #include is a preprocessor directive using which we import header files.
Syntax:
#include <library_name>
To know more about header files, please refer to the article – Header Files in C/C++.
2. Namespace
A namespace in C++ is used to provide a scope or a region where we define identifiers. It is used to avoid name conflicts between two identifiers as only unique names can be used as identifiers.
In line #2, we have used the using namespace std statement for specifying that we will be the standard namespace where all the standard library functions are defined.
Syntax:
using namespace std;
To know more about namespaces in C++, please refer to the article – Namespaces in C++.
3. Main Function
Functions are basic building blocks of a C++ program that contains the instructions for performing some specific task. Apart from the instructions present in its body, a function definition also contains information about its return type and parameters. To know more about C++ functions, please refer to the article Functions in C++.
In line #3, we defined the main function as int main(). The main function is the most important part of any C++ program. The program execution always starts from the main function. All the other functions are called from the main function. In C++, the main function is required to return some value indicating the execution status.
Syntax:
int main() { ... code .... return 0; }
4. Blocks
Blocks are the group of statements that are enclosed within { } braces. They define the scope of the identifiers and are generally used to enclose the body of functions and control statements.
The body of the main function is from line #4 to line #9 enclosed within { }.
Syntax:
{ // Body of the Function return 0; }
5. Semicolons
As you may have noticed by now, each statement in the above code is followed by a ( ; ) semicolon symbol. It is used to terminate each line of the statement of the program. When the compiler sees this semicolon, it terminates the operation of that line and moves to the next line.
Syntax:
any_statement ;
6. Identifiers
We use identifiers for the naming of variables, functions, and other user-defined data types. An identifier may consist of uppercase and lowercase alphabetical characters, underscore, and digits. The first letter must be an underscore or an alphabet.
Example:
int num1 = 24; int num2 = 34;
num1 & num2 are the identifiers and int is the data type.
7. Keywords
In the C++ programming language, there are some reserved words that are used for some special meaning in the C++ program. It can’t be used for identifiers.
For example, the words int, return, and using are some keywords used in our program. These all have some predefined meaning in the C++ language.
There are total 95 keywords in C++. These are some keywords.
int void if while for auto bool break this static new true false case char class
To know more about Identifiers and Keywords in C++, refer to the article C/C++ Tokens.
8. Basic Output cout
In line #7, we have used the cout method which is the basic output method in C++ to output the sum of two numbers in the standard output stream (stdout).
Syntax:
cout << result << endl;
To know more about basic input and output in C++, please refer to the article – Basic Input and Output in C.
Now, we have a better understanding of the basic syntax structure of the above C++ program. Let’s try to execute this program and see if it works correctly.
- C++
58
The above program runs correctly and displays the specified output because we have followed the syntax rules of C++. We will learn more about these basic elements later.
Object-Oriented Programming in C++
C++ programming language supports both procedural-oriented and object-oriented programming. The above example is based on the procedural-oriented programming paradigm. So let’s take another example to discuss Object Oriented Programming in C++.
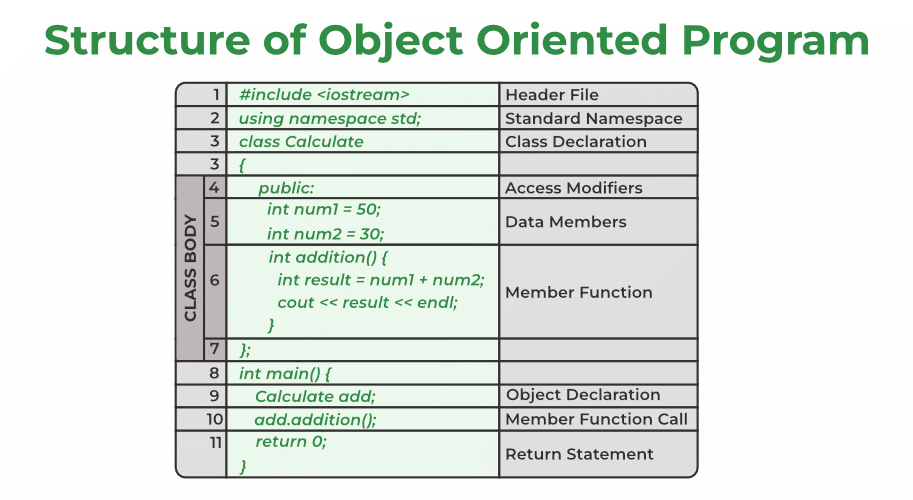
1. Class
A class is a template of an object. For example, the animal is a class & dog is the object of the animal class. It is a user-defined data type. A class has its own attributes (data members) and behavior (member functions). The first letter of the class name is always capitalized & use the class keyword for creating the class.
In line #3, we have declared a class named Calculate and its body expands from line #3 to line #7.
Syntax:
class class_name{ // class body };
To know more about classes in C++, please refer to this article.
2. Data Members & Member Functions
The attributes or data in the class are defined by the data members & the functions that work on these data members are called the member functions.
Example:
class Calculate{ public: int num1 = 50; // data member int num2 = 30; // data member // member function int addition() { int result = num1 + num2; cout << result << endl; } };
In the above example, num1 and num2 are the data member & addition() is a member function that is working on these two data members. There is a keyword here public that is access modifiers. The access modifier decides who has access to these data members & member functions. public access modifier means these data members & member functions can get access by anyone.
3. Object
The object is an instance of a class. The class itself is just a template that is not allocated any memory. To use the data and methods defined in the class, we have to create an object of that class.
They are declared in the similar way we declare variables in C++.
Syntax:
class_name object_name;
We use dot operator ( . ) for accessing data and methods of an object.
To know more about objects in C++, please refer to the article – C++ Classes and Objects.
Now, let’s execute our code to see the output of the program.
- C++
80
C++ Comments
Comments in C++ are meant to explain the code as well as to make it more readable. When testing alternative code, it can also be used to prevent execution. The purpose of the comments is to provide information about code lines. Programmers commonly use comments to document their work.
Why Comments are used in C++?
Comments in C++ are used to summarize an algorithm, identify a variable’s purpose, or clarify a code segment that appears unclear. Comments are also used for:
- Comments are used for easier debugging.
- It makes a program more readable and gives an overall description of the code.
- Comments are helpful in skipping the execution of some parts of the code.
- Every time a program or code is reused after long periods of time, the comment recaps all the information of the code quickly.
Types of Comments in C++
In C++ there are two types of comments in C++:
- Single-line comment
- Multi-line comment
1. Single Line Comment
In C++ Single line comments are represented as // double forward slash. It applies comments to a single line only. The compiler ignores any text after // and it will not be executed.
Syntax:
// Single line commentExample:
C++
OutputGFG!2. Multi-Line Comment
A multi-line comment can occupy many lines of code, it starts with /* and ends with */, but it cannot be nested. Any text between /* and */ will be ignored by the compiler.
Syntax:
/* Multiline Comment . . . */Example:
C++
OutputGFG!
C++ Keywords
C++ is a powerful language. In C++, we can write structured programs and object-oriented programs also. C++ is a superset of C and therefore most constructs of C are legal in C++ with their meaning unchanged. However, there are some exceptions and additions.
C++ is a powerful language. In C++, we can write structured programs and object-oriented programs also. C++ is a superset of C and therefore most constructs of C are legal in C++ with their meaning unchanged. However, there are some exceptions and additions.
Token
When the compiler is processing the source code of a C++ program, each group of characters separated by white space is called a token. Tokens are the smallest individual units in a program. A C++ program is written using tokens. It has the following tokens:
When the compiler is processing the source code of a C++ program, each group of characters separated by white space is called a token. Tokens are the smallest individual units in a program. A C++ program is written using tokens. It has the following tokens:
Keywords
Keywords(also known as reserved words) have special meanings to the C++ compiler and are always written or typed in short(lower) cases. Keywords are words that the language uses for a special purpose, such as void, int, public, etc. It can’t be used for a variable name or function name or any other identifiers. The total count of reserved keywords is 95. Below is the table for some commonly used C++ keywords.
C++ Keyword
asm double new switch auto else operator template break enum private this case extern protected throw catch float public try char for register typedef class friend return union const goto short unsigned continue if signed virtual default inline sizeof void delete int static volatile do long struct while
Note: The keywords not found in ANSI C are shown here in boldface.
- asm: To declare that a block of code is to be passed to the assembler.
- auto: A storage class specifier that is used to define objects in a block.
- break: Terminates a switch statement or a loop.
- case: Used specifically within a switch statement to specify a match for the statement’s expression.
- catch: Specifies actions taken when an exception occurs.
- char: Fundamental data type that defines character objects.
- class: To declare a user-defined type that encapsulates data members and operations or member functions.
- const: To define objects whose value will not alter throughout the lifetime of program execution.
- continue:- Transfers control to the start of a loop.
- default:- Handles expression values in a switch statement that are not handled by case.
- delete: Memory deallocation operator.
- do: indicate the start of a do-while statement in which the sub-statement is executed repeatedly until the value of the expression is logical-false.
- double: Fundamental data type used to define a floating-point number.
- else: Used specifically in an if-else statement.
- enum: To declare a user-defined enumeration data type.
- extern: An identifier specified as an extern has an external linkage to the block.
- float:- Fundamental data type used to define a floating-point number.
- for: Indicates the start of a statement to achieve repetitive control.
- friend: A class or operation whose implementation can access the private data members of a class.
- goto: Transfer control to a specified label.
- if: Indicate the start of an if statement to achieve selective control.
- inline: A function specifier that indicates to the compiler that inline substitution of the function body is to be preferred to the usual function call implementation.
- int: Fundamental data type used to define integer objects.
- long: A data type modifier that defines a 32-bit int or an extended double.
- new: Memory allocation operator.
- operator: Overloads a c++ operator with a new declaration.
- private: Declares class members which are not visible outside the class.
- protected: Declares class members which are private except to derived classes
- public: Declares class members who are visible outside the class.
- register: A storage class specifier that is an auto specifier, but which also indicates to the compiler that an object will be frequently used and should therefore be kept in a register.
- return: Returns an object to a function’s caller.
- short: A data type modifier that defines a 16-bit int number.
- signed: A data type modifier that indicates an object’s sign is to be stored in the high-order bit.
- sizeof: Returns the size of an object in bytes.
- static: The lifetime of an object-defined static exists throughout the lifetime of program execution.
- struct: To declare new types that encapsulate both data and member functions.
- switch: This keyword is used in the “Switch statement”.
- template: parameterized or generic type.
- this: A class pointer points to an object or instance of the class.
- throw: Generate an exception.
- try: Indicates the start of a block of exception handlers.
- typedef: Synonym for another integral or user-defined type.
- union: Similar to a structure, struct, in that it can hold different types of data, but a union can hold only one of its members at a given time.
- unsigned: A data type modifier that indicates the high-order bit is to be used for an object.
- virtual: A function specifier that declares a member function of a class that will be redefined by a derived class.
- void: Absent of a type or function parameter list.
- volatile: Define an object which may vary in value in a way that is undetectable to the compiler.
- while: Start of a while statement and end of a do-while statement.
Keywords(also known as reserved words) have special meanings to the C++ compiler and are always written or typed in short(lower) cases. Keywords are words that the language uses for a special purpose, such as void, int, public, etc. It can’t be used for a variable name or function name or any other identifiers. The total count of reserved keywords is 95. Below is the table for some commonly used C++ keywords.
C++ Keyword | |||
---|---|---|---|
asm | double | new | switch |
auto | else | operator | template |
break | enum | private | this |
case | extern | protected | throw |
catch | float | public | try |
char | for | register | typedef |
class | friend | return | union |
const | goto | short | unsigned |
continue | if | signed | virtual |
default | inline | sizeof | void |
delete | int | static | volatile |
do | long | struct | while |
Note: The keywords not found in ANSI C are shown here in boldface.
- asm: To declare that a block of code is to be passed to the assembler.
- auto: A storage class specifier that is used to define objects in a block.
- break: Terminates a switch statement or a loop.
- case: Used specifically within a switch statement to specify a match for the statement’s expression.
- catch: Specifies actions taken when an exception occurs.
- char: Fundamental data type that defines character objects.
- class: To declare a user-defined type that encapsulates data members and operations or member functions.
- const: To define objects whose value will not alter throughout the lifetime of program execution.
- continue:- Transfers control to the start of a loop.
- default:- Handles expression values in a switch statement that are not handled by case.
- delete: Memory deallocation operator.
- do: indicate the start of a do-while statement in which the sub-statement is executed repeatedly until the value of the expression is logical-false.
- double: Fundamental data type used to define a floating-point number.
- else: Used specifically in an if-else statement.
- enum: To declare a user-defined enumeration data type.
- extern: An identifier specified as an extern has an external linkage to the block.
- float:- Fundamental data type used to define a floating-point number.
- for: Indicates the start of a statement to achieve repetitive control.
- friend: A class or operation whose implementation can access the private data members of a class.
- goto: Transfer control to a specified label.
- if: Indicate the start of an if statement to achieve selective control.
- inline: A function specifier that indicates to the compiler that inline substitution of the function body is to be preferred to the usual function call implementation.
- int: Fundamental data type used to define integer objects.
- long: A data type modifier that defines a 32-bit int or an extended double.
- new: Memory allocation operator.
- operator: Overloads a c++ operator with a new declaration.
- private: Declares class members which are not visible outside the class.
- protected: Declares class members which are private except to derived classes
- public: Declares class members who are visible outside the class.
- register: A storage class specifier that is an auto specifier, but which also indicates to the compiler that an object will be frequently used and should therefore be kept in a register.
- return: Returns an object to a function’s caller.
- short: A data type modifier that defines a 16-bit int number.
- signed: A data type modifier that indicates an object’s sign is to be stored in the high-order bit.
- sizeof: Returns the size of an object in bytes.
- static: The lifetime of an object-defined static exists throughout the lifetime of program execution.
- struct: To declare new types that encapsulate both data and member functions.
- switch: This keyword is used in the “Switch statement”.
- template: parameterized or generic type.
- this: A class pointer points to an object or instance of the class.
- throw: Generate an exception.
- try: Indicates the start of a block of exception handlers.
- typedef: Synonym for another integral or user-defined type.
- union: Similar to a structure, struct, in that it can hold different types of data, but a union can hold only one of its members at a given time.
- unsigned: A data type modifier that indicates the high-order bit is to be used for an object.
- virtual: A function specifier that declares a member function of a class that will be redefined by a derived class.
- void: Absent of a type or function parameter list.
- volatile: Define an object which may vary in value in a way that is undetectable to the compiler.
- while: Start of a while statement and end of a do-while statement.
What is the identifier?
Identifiers refer to the name of variables, functions, arrays, classes, etc. created by the programmer. They are the fundamental requirement of any language.
Rules for naming identifiers:
- Identifier names can not start with a digit or any special character.
- A keyword cannot be used as s identifier name.
- Only alphabetic characters, digits, and underscores are permitted.
- The upper case and lower case letters are distinct. i.e., A and a are different in C++.
- The valid identifiers are GFG, gfg, and geeks_for_geeks.
Examples of good and bad identifiers
Invalid Identifier Bad Identifier Good Identifier Cash prize C_prize cashprize catch catch_1 catch1 1list list_1 list1
Example:
Identifiers refer to the name of variables, functions, arrays, classes, etc. created by the programmer. They are the fundamental requirement of any language.
Rules for naming identifiers:
- Identifier names can not start with a digit or any special character.
- A keyword cannot be used as s identifier name.
- Only alphabetic characters, digits, and underscores are permitted.
- The upper case and lower case letters are distinct. i.e., A and a are different in C++.
- The valid identifiers are GFG, gfg, and geeks_for_geeks.
Examples of good and bad identifiers
Invalid Identifier | Bad Identifier | Good Identifier |
Cash prize | C_prize | cashprize |
catch | catch_1 | catch1 |
1list | list_1 | list1 |
Example:
C++
OutputIdentifier result is: 1
Identifier result is: 1
How keywords are different from identifiers?
So there are some main properties of keywords that distinguish keywords from identifiers:
Keywords Identifiers Keywords are predefined/reserved words identifiers are the values used to define different programming items like a variable, integers, structures, and unions. Keywords always start in lowercase identifiers can start with an uppercase letter as well as a lowercase letter. It defines the type of entity. It classifies the name of the entity. A keyword contains only alphabetical characters, an identifier can consist of alphabetical characters, digits, and underscores. It should be lowercase. It can be both upper and lowercase. No special symbols or punctuations are used in keywords and identifiers. No special symbols or punctuations are used in keywords and identifiers. The only underscore can be used in an identifier. Keywords: int, char, while, do. Identifiers: Geeks_for_Geeks, GFG, Gfg1.
Example: Below is the program for how to use different keywords in the program:
So there are some main properties of keywords that distinguish keywords from identifiers:
Keywords | Identifiers |
Keywords are predefined/reserved words | identifiers are the values used to define different programming items like a variable, integers, structures, and unions. |
Keywords always start in lowercase | identifiers can start with an uppercase letter as well as a lowercase letter. |
It defines the type of entity. | It classifies the name of the entity. |
A keyword contains only alphabetical characters, | an identifier can consist of alphabetical characters, digits, and underscores. |
It should be lowercase. | It can be both upper and lowercase. |
No special symbols or punctuations are used in keywords and identifiers. | No special symbols or punctuations are used in keywords and identifiers. The only underscore can be used in an identifier. |
Keywords: int, char, while, do. | Identifiers: Geeks_for_Geeks, GFG, Gfg1. |
Example: Below is the program for how to use different keywords in the program:
C++
OutputC++
C++
C++ Variables
Variables in C++ is a name given to a memory location. It is the basic unit of storage in a program.
- The value stored in a variable can be changed during program execution.
- A variable is only a name given to a memory location, all the operations done on the variable effects that memory location.
- In C++, all the variables must be declared before use.
Variables in C++ is a name given to a memory location. It is the basic unit of storage in a program.
- The value stored in a variable can be changed during program execution.
- A variable is only a name given to a memory location, all the operations done on the variable effects that memory location.
- In C++, all the variables must be declared before use.
How to Declare Variables?
A typical variable declaration is of the form:
// Declaring a single variable
type variable_name;
// Declaring multiple variables:
type variable1_name, variable2_name, variable3_name;
A variable name can consist of alphabets (both upper and lower case), numbers, and the underscore ‘_’ character. However, the name must not start with a number.
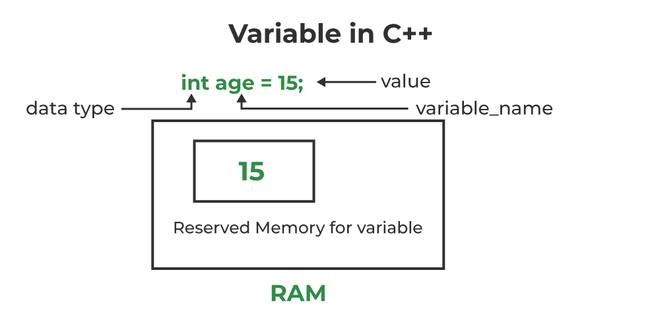
Initialization of a variable in C++
In the above diagram,
datatype: Type of data that can be stored in this variable.
variable_name: Name given to the variable.
value: It is the initial value stored in the variable.
Examples:
// Declaring float variable
float simpleInterest;
// Declaring integer variable
int time, speed;
// Declaring character variable
char var;
A typical variable declaration is of the form:
// Declaring a single variable type variable_name; // Declaring multiple variables: type variable1_name, variable2_name, variable3_name;A variable name can consist of alphabets (both upper and lower case), numbers, and the underscore ‘_’ character. However, the name must not start with a number.
Initialization of a variable in C++
In the above diagram,
datatype: Type of data that can be stored in this variable.
variable_name: Name given to the variable.
value: It is the initial value stored in the variable.Examples:
// Declaring float variable float simpleInterest; // Declaring integer variable int time, speed; // Declaring character variable char var;
We can also provide values while declaring the variables as given below:
int a=50,b=100; //declaring 2 variable of integer type
float f=50.8; //declaring 1 variable of float type
char c='Z'; //declaring 1 variable of char type
int a=50,b=100; //declaring 2 variable of integer type float f=50.8; //declaring 1 variable of float type char c='Z'; //declaring 1 variable of char type
Rules For Declaring Variable
- The name of the variable contains letters, digits, and underscores.
- The name of the variable is case sensitive (ex Arr and arr both are different variables).
- The name of the variable does not contain any whitespace and special characters (ex #,$,%,*, etc).
- All the variable names must begin with a letter of the alphabet or an underscore(_).
- We cannot used C++ keyword(ex float,double,class)as a variable name.
Valid variable names:
int x; //can be letters
int _yz; //can be underscores
int z40;//can be letters
Invalid variable names:
int 89; Should not be a number
int a b; //Should not contain any whitespace
int double;// C++ keyword CAN NOT BE USED
- The name of the variable contains letters, digits, and underscores.
- The name of the variable is case sensitive (ex Arr and arr both are different variables).
- The name of the variable does not contain any whitespace and special characters (ex #,$,%,*, etc).
- All the variable names must begin with a letter of the alphabet or an underscore(_).
- We cannot used C++ keyword(ex float,double,class)as a variable name.
Valid variable names:
int x; //can be letters int _yz; //can be underscores int z40;//can be lettersInvalid variable names:
int 89; Should not be a number int a b; //Should not contain any whitespace int double;// C++ keyword CAN NOT BE USED
Difference Between Variable Declaration and Definition
The variable declaration refers to the part where a variable is first declared or introduced before its first use. A variable definition is a part where the variable is assigned a memory location and a value. Most of the time, variable declaration and definition are done together.
See the following C++ program for better clarification:
The variable declaration refers to the part where a variable is first declared or introduced before its first use. A variable definition is a part where the variable is assigned a memory location and a value. Most of the time, variable declaration and definition are done together.
See the following C++ program for better clarification:
C++
Outputa
Time Complexity: O(1)
Space Complexity: O(1)
OutputaTime Complexity: O(1)
Space Complexity: O(1)
Types of Variables
There are three types of variables based on the scope of variables in C++
- Local Variables
- Instance Variables
- Static Variables
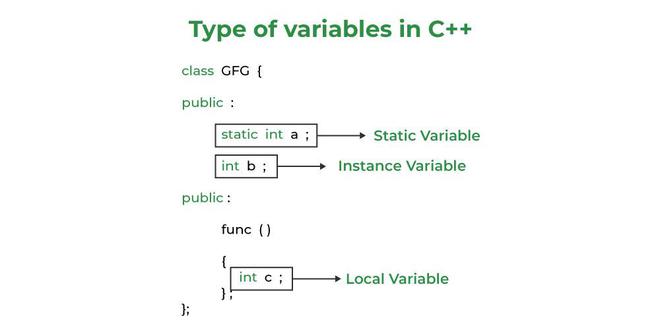
Types of Variables in C++
Let us now learn about each one of these variables in detail.
- Local Variables: A variable defined within a block or method or constructor is called a local variable.
- These variables are created when entered into the block or the function is called and destroyed after exiting from the block or when the call returns from the function.
- The scope of these variables exists only within the block in which the variable is declared. i.e. we can access this variable only within that block.
- Initialization of Local Variable is Mandatory.
- Instance Variables: Instance variables are non-static variables and are declared in a class outside any method, constructor, or block.
- As instance variables are declared in a class, these variables are created when an object of the class is created and destroyed when the object is destroyed.
- Unlike local variables, we may use access specifiers for instance variables. If we do not specify any access specifier then the default access specifier will be used.
- Initialization of Instance Variable is not Mandatory.
- Instance Variable can be accessed only by creating objects.
- Static Variables: Static variables are also known as Class variables.
- These variables are declared similarly as instance variables, the difference is that static variables are declared using the static keyword within a class outside any method constructor or block.
- Unlike instance variables, we can only have one copy of a static variable per class irrespective of how many objects we create.
- Static variables are created at the start of program execution and destroyed automatically when execution ends.
- Initialization of Static Variable is not Mandatory. Its default value is 0
- If we access the static variable like the Instance variable (through an object), the compiler will show the warning message and it won’t halt the program. The compiler will replace the object name with the class name automatically.
- If we access the static variable without the class name, the Compiler will automatically append the class name.
There are three types of variables based on the scope of variables in C++
- Local Variables
- Instance Variables
- Static Variables
Types of Variables in C++
Let us now learn about each one of these variables in detail.
- Local Variables: A variable defined within a block or method or constructor is called a local variable.
- These variables are created when entered into the block or the function is called and destroyed after exiting from the block or when the call returns from the function.
- The scope of these variables exists only within the block in which the variable is declared. i.e. we can access this variable only within that block.
- Initialization of Local Variable is Mandatory.
- Instance Variables: Instance variables are non-static variables and are declared in a class outside any method, constructor, or block.
- As instance variables are declared in a class, these variables are created when an object of the class is created and destroyed when the object is destroyed.
- Unlike local variables, we may use access specifiers for instance variables. If we do not specify any access specifier then the default access specifier will be used.
- Initialization of Instance Variable is not Mandatory.
- Instance Variable can be accessed only by creating objects.
- Static Variables: Static variables are also known as Class variables.
- These variables are declared similarly as instance variables, the difference is that static variables are declared using the static keyword within a class outside any method constructor or block.
- Unlike instance variables, we can only have one copy of a static variable per class irrespective of how many objects we create.
- Static variables are created at the start of program execution and destroyed automatically when execution ends.
- Initialization of Static Variable is not Mandatory. Its default value is 0
- If we access the static variable like the Instance variable (through an object), the compiler will show the warning message and it won’t halt the program. The compiler will replace the object name with the class name automatically.
- If we access the static variable without the class name, the Compiler will automatically append the class name.
Instance Variable Vs Static Variable
- Each object will have its own copy of the instance variable whereas We can only have one copy of a static variable per class irrespective of how many objects we create.
- Changes made in an instance variable using one object will not be reflected in other objects as each object has its own copy of the instance variable. In the case of static, changes will be reflected in other objects as static variables are common to all objects of a class.
- We can access instance variables through object references and Static Variables can be accessed directly using the class name.
- The syntax for static and instance variables:
class Example
{
static int a; // static variable
int b; // instance variable
}
- Each object will have its own copy of the instance variable whereas We can only have one copy of a static variable per class irrespective of how many objects we create.
- Changes made in an instance variable using one object will not be reflected in other objects as each object has its own copy of the instance variable. In the case of static, changes will be reflected in other objects as static variables are common to all objects of a class.
- We can access instance variables through object references and Static Variables can be accessed directly using the class name.
- The syntax for static and instance variables:
class Example { static int a; // static variable int b; // instance variable }
C++ introduces the concept of classes and objects, allowing you to organize code around real-world entities and their interactions.
Classes define the structure and behavior of objects, encapsulating data and methods into a single unit.
Inheritance, polymorphism, and encapsulation are key principles of OOP supported by C++.
Standard Template Library (STL):
C++ provides a rich library known as the Standard Template Library (STL) that includes containers (like vectors, lists, maps), algorithms (sorting, searching), and other utility classes.
STL components enhance productivity by offering pre-built data structures and algorithms.
Function Overloading and Default Arguments:
C++ allows functions to have multiple implementations based on their parameters. This is called function overloading.
Default arguments can be provided to functions, reducing the need for multiple function definitions.
Operator Overloading:
C++ lets you redefine the behavior of operators for custom classes, allowing more intuitive interactions with objects.
Dynamic Memory Management:
C++ retains the manual memory management features of C.
It also introduces features like new and delete operators for dynamic memory allocation and deallocation.
Namespaces:
C++ introduces namespaces to prevent naming conflicts and organize code into separate logical units.
Exception Handling:
C++ provides mechanisms for handling exceptions and errors more gracefully than C's traditional error codes.
Type Casting:
C++ offers more flexible type casting options, including both C-style casting and safer alternatives like dynamic_cast.
References:
C++ includes references as an alternative to pointers, allowing more convenient and safer access to variables.
STL Iterators:
Iterators in the STL provide a way to access elements in containers in a standardized manner, abstracting away the underlying container type.
RAII (Resource Acquisition Is Initialization):
C++ encourages the use of RAII, a design pattern where resource management (like memory) is tied to the lifespan of objects.
Standardization:
C++ has evolved through various versions, with standards like C++98, C++11, C++14, C++17, and C++20 introducing new features and improvements.
0 Comments